Heap Exploitation
2024-01-01 Back to posts
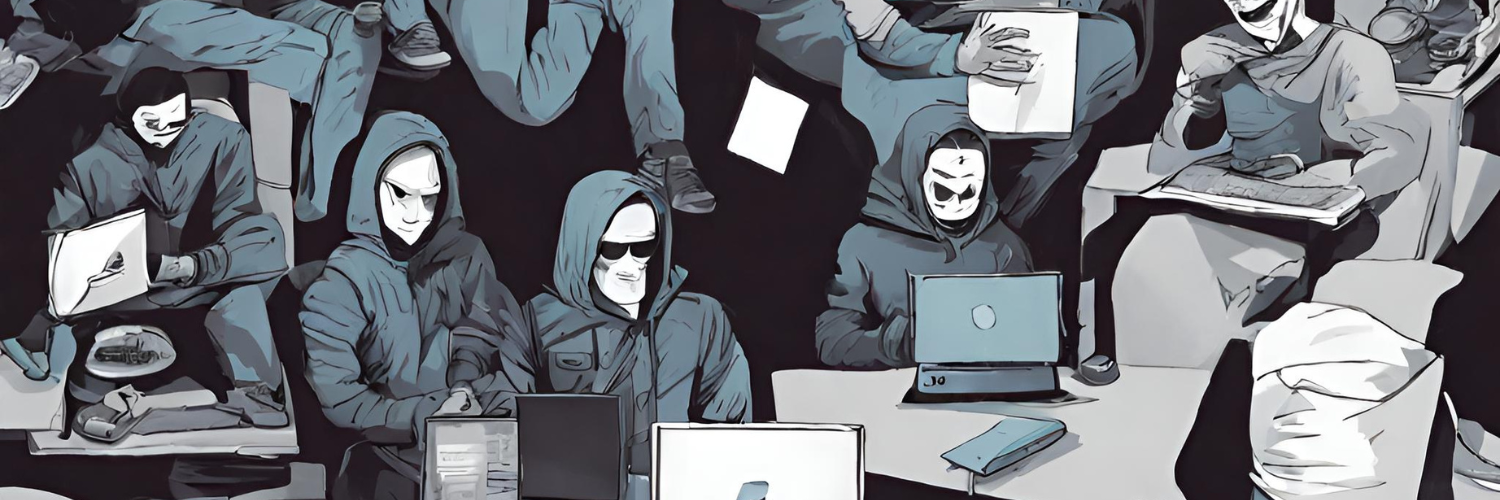
Fancy way to exploit the heap
Introduction
The heap is a memory region used for dynamic memory allocation in programs. It is a common target for attackers looking to exploit memory corruption vulnerabilities. In this post, we will explore how heap exploitation works and how attackers can leverage heap vulnerabilities to gain unauthorized access to a system.
In this post, we will focus on glibc
heap exploitation, which is a common target for attackers due to its widespread use in Linux systems. We will cover the basics of heap memory management, common heap exploitation techniques, and how to protect against heap vulnerabilities.
Heap Memory Management
The heap is a memory region used for dynamic memory allocation in programs. When a program requests memory from the heap using functions like malloc
or free
, the memory manager allocates or deallocates memory blocks from the heap. The memory manager keeps track of the allocated and free memory blocks using data structures like bins, chunks, and arenas.
Basic Vulnerabilities
Heap vulnerabilities can arise from memory corruption bugs like buffer overflows, use-after-free, double-free, and other memory safety issues. These vulnerabilities can be exploited to overwrite data structures in the heap, control the program’s execution flow, or leak sensitive information from memory.
Example: double-free vulnerability
#include <stdlib.h>
int main() {
int *ptr = (int *)malloc(sizeof(int));
free(ptr);
some_function_by_which_the_user_can_allocate_stuff();
free(ptr); // Double-free vulnerability
return 0;
}
In the code above, we have a double-free vulnerability where the same memory block is freed twice. This can corrupt the heap’s data structures and lead to undefined behavior. Attackers can exploit this vulnerability to manipulate the heap’s metadata and gain control of the program. In fact, after the first free, the chunk goes into the fastbin list. If we allocate a chunk of the same size, we can get the same chunk back, modify it to point to a fake chunk (which we want to control), and then free it again. This is a common technique used in heap exploitation called fastbin attack.
Conclusion
Heap exploitation is a complex topic that requires a deep understanding of memory management and memory corruption vulnerabilities. By understanding how heap memory management works and common heap exploitation techniques, developers can better protect their software from heap vulnerabilities. It is important to follow secure coding practices, use memory-safe languages, and perform security testing to identify and fix heap vulnerabilities in software.